-
Notifications
You must be signed in to change notification settings - Fork 3
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Lot's of G0 moves in GCODE #45
Comments
Found a lib that can split concave polygons in to convex polygons (minimum convex decomposition) https://www.npmjs.com/package/poly-decomp (no support for holes) paper on the subject https://www.sciencedirect.com/science/article/pii/S0925772105001008 |
I've written an alternate aproach that can be seen here |
When combing is turned on there are some times a lot of unneeded G0 moves.
With combing turned ON
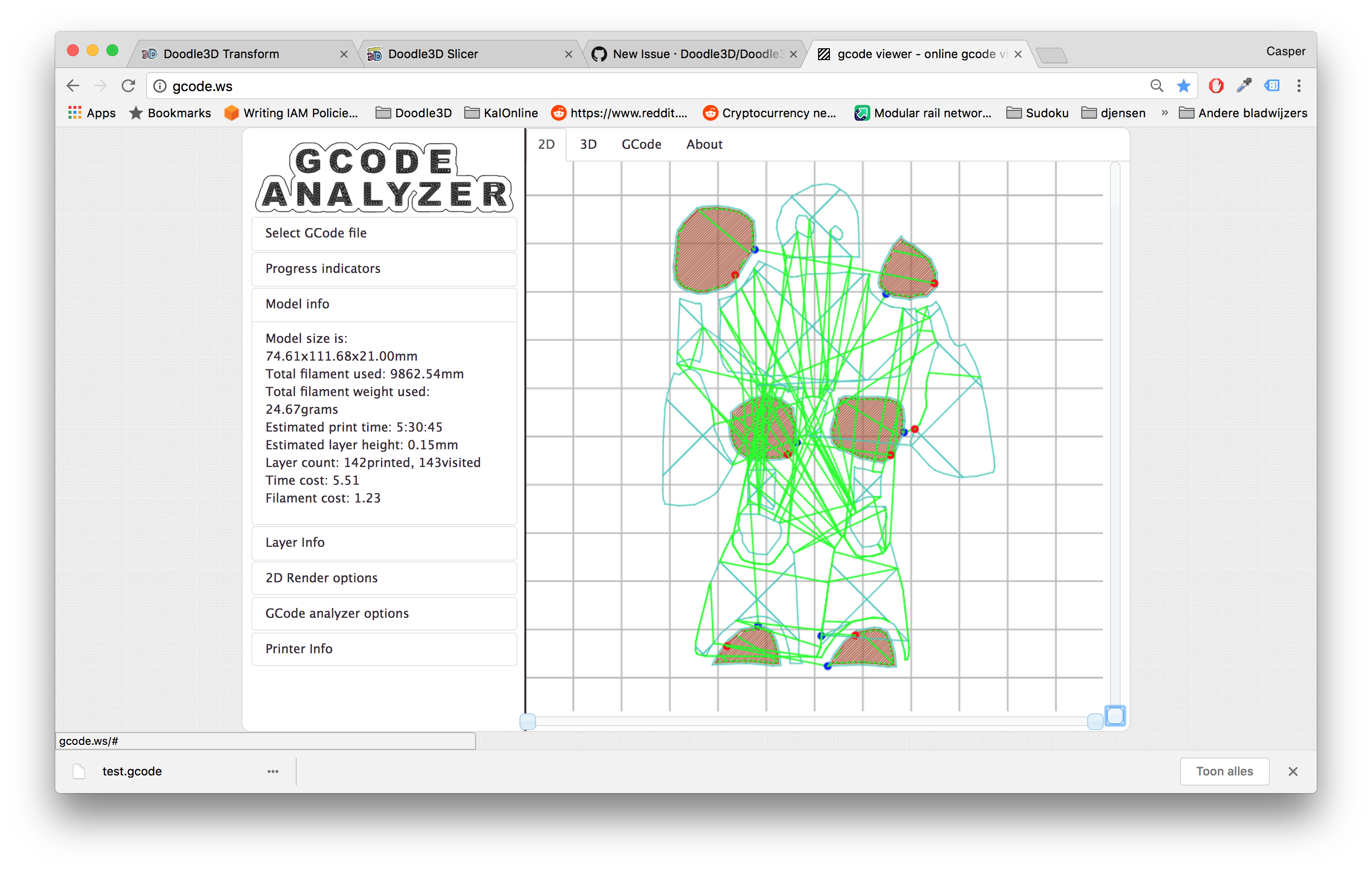
With combing turned OFF
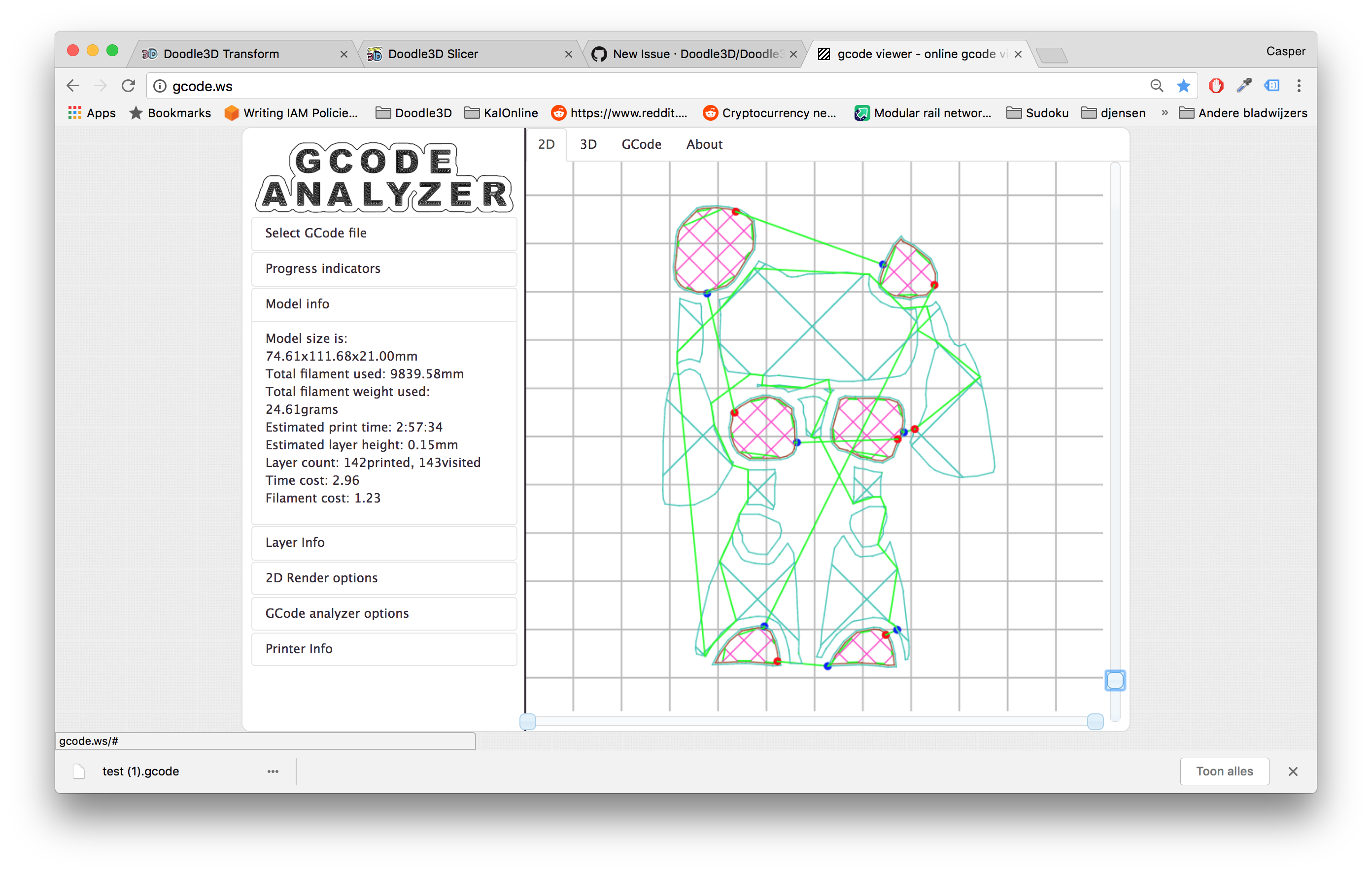
The text was updated successfully, but these errors were encountered: